Getting Started
Install
- npm
- yarn
- pnpm
- bun
npm i --save-dev mocq
yarn add --dev mocq
pnpm add --save-dev mocq
bun add --dev mocq
Basic Concepts
[mocq]
is a super small (<9 kB unpacked
) utility
for data creation, connection & execution coordination
[mocq]
exports a single function mocq
, this function takes in a custom config object, vaildates the configuration and returns two functions generate & execute
import { mocq } from 'mocq';
/* ... */
const { generate, execute } = mocq(customConfigObj);
Configuration
[mocq]
is configured with an object of key-value pairs
where the:
key
is a contextual string that you choose
- you will use this key to reference resolved data from
connections
of other key-value pairs
value
is a MocQ config object
- user defined functions:
- how to generate a data instance
- how to connect to other keys in the configuration
- how to handle data when execute is called
Each key should correspond to a single data object type
import { mocq } from 'mocq'
const customConfigObj = {
myCustomKey: {
generator: // a function to create a myCustomKey node
count: // the number of myCustomKey nodes wanted
connections?: // ability to hook into resolved data from other keys in config & amend myCustomKey data
handler?: // ability to execute functions against myCustomKey resolved data
},
myCustomKey2: {
/* ... */
},
/* ... */
}
const { generate, execute } = mocq(customConfigObj)
The order of keys in the config does not matter, [mocq]
manages the execution order
Go through the Tutorial, checkout the API or browse some Examples for more information on configuration
Data Resolution
Use connections to amend generated data with data generated via another key in the top level configuration
Data is fully resolved before being passed to a connection function
meaning the data you're handed in a connection function is the same data that would come back under the same key from the
generate
function
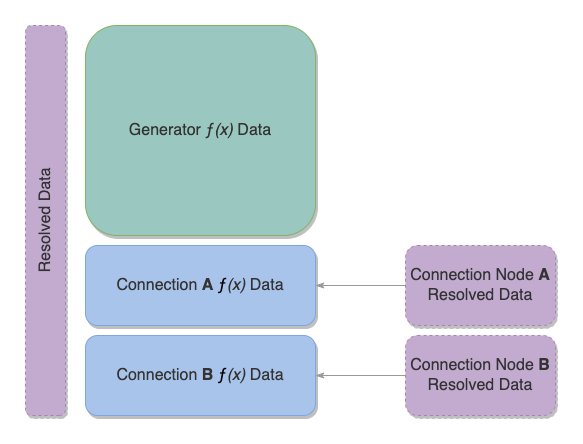
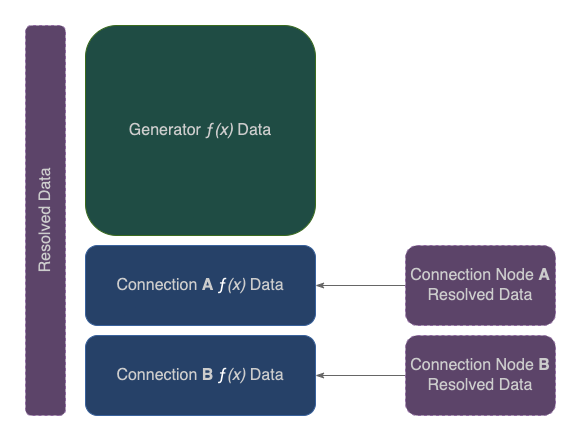
Data is resolved by spreading the top level keys of the return of connection functions over the return of generator function
{
...generatorFn(),
...connectionFn1(),
...connectionFn2(),
...connectionFnx(),
}
Execution Order
[mocq]
derives the execution order from connections, cyclic dependencies & self reference will cause configuration validation to fail
handler
functions allow you utilize the execution order to invoke functions on the generated data
this is typically where you'd use your
src
code to move data into a data store (database operations, api calls, etc.), the execution order ensures dependent data is there at time of execution allowing data to be stitched together on the fly
generate
sync function that generates and connects data
returns resolved generated data
const { data: { key1, key2, ..., keyx } } = generate()
execute
async function that generates and connects data & executes handler functions
returns resolved generated data
const { data: { key1, key2, ..., keyx } } = await execute()